3 Ways to Reuse Twig Templates in Symfony. In this blog, we will talk about Symfony and how we can reuse Symfony templates. Symfony provides an awesome template engine called Twig. Twig is an open-source framework maintained by Fabien Potencier, and licensed under the BSD license. The first version of this framework was made by Armin Ronacher. Symfony's Workflow component allows you to define a life cycle your object can go through with its statuses. Each step your object can go through is called a place, with the transitions defining the action the object needs to take to get from one place to another.
In this blog, we will talk about Symfony and how we can reuse Symfony templates. Symfony provides an awesome template engine called Twig. Twig is an open-source framework maintained by Fabien Potencier, and licensed under the BSD license. The first version of this framework was made by Armin Ronacher.
What is Twig?
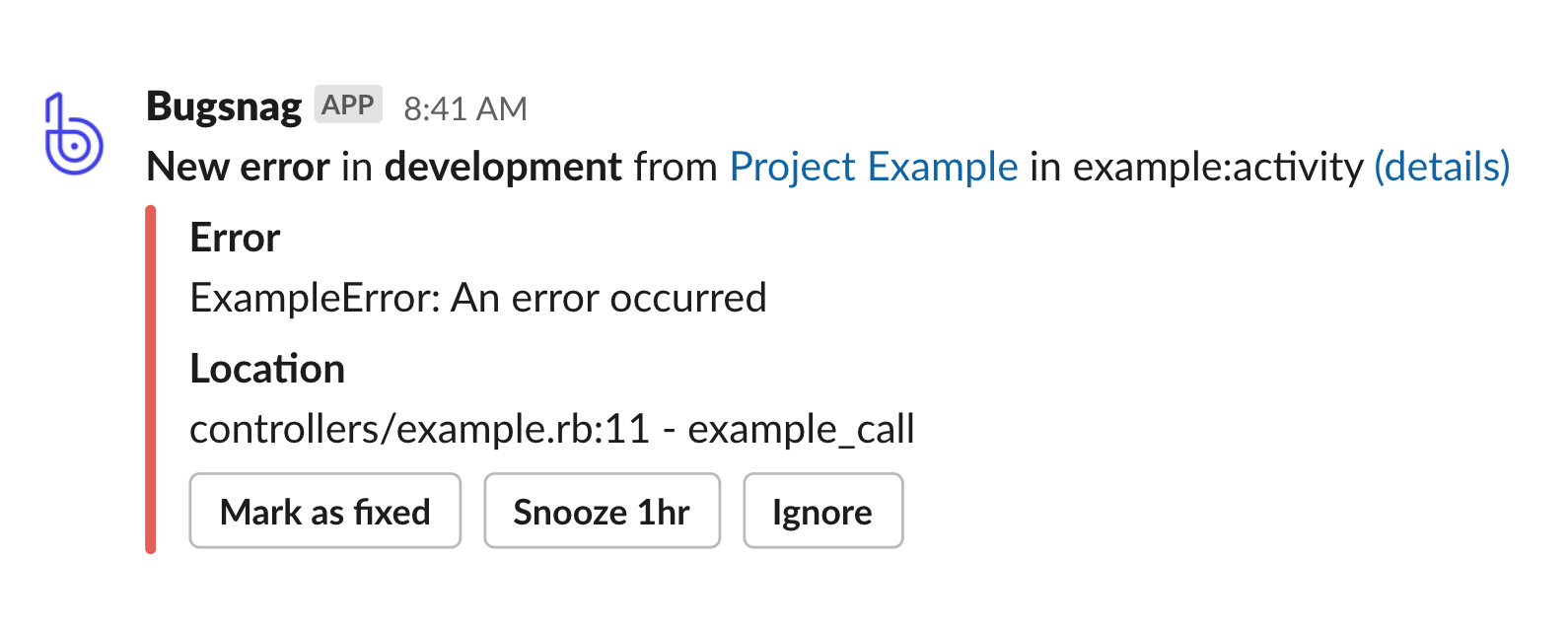
Twig, which is founded by Symfony, is a modern template engine for PHP. Twig directly compiles the templates into plain PHP code. So the effort of converting into PHP is reduced very much. Like most languages, Twig has a sandbox that protects us from untrusted and suspicious code. Hence making us use Twig as the template language and it makes Twig more secure. Developers can create their own custom tasks, filters, and DSL too. The output format of Twig is mainly HTML but it can output various text formats as well.
Many open-source projects like Symfony, Drupal 8, eZPublish, PhpBB, Matomo, OroCRM, use Twig as a template engine. Frameworks in which Twig is supported are Slim, Yii, Laravel, and CodeIgniter, etc.
`{{..}}`
. For logic, such as conditional statements or loops, it uses curly brace percentage `
{%..%}`
. It uses curly brace hash `{#..#}`
for comments, comments are not rendered on the screen as they are not considered as part of code during compilation time.Twig is supported in many integrated development environments(IDEs) like Eclipse, Komodo, Komodo edit, Netbeans with Twig syntax plugin, and PhpStorm. Various text editors that are used by developers for Twig, with Twig extension, are Atom, emacs, Notepad++, Sublime Text, Visual Studio Code, Brackets, vim, TextMate, GTK source view, Coda, Coda 2, SubEthaEdit.
An example code for Twig is given below:
`
for`
tag to create a loop. Other than `for`
tags we have used other tags also like `extends`
, `block`
, etc. Twig code consists of tags, filters, functions, operators, and tests. In Symfony, functions defined by Symfony and Twig filters can also be used and you can even define your own Twig filters and functions.Basic use
Twig supports two kinds of delimiters:
{%..%}
: It is used for executing conditional and iterative statements.{{..}}
: It is used for printing statements and variables.Variable: For getting variable in Twig, either for an Object or array,
And for setting the value of variables we can do:
Filter: Filters like sort can be used like:
Functions: Functions supported by Twig are used by:
Comment: Comments are used for making code more readable and understandable. In Twig we can use comments as:
Macros: When we have to reuse a markup HTML to avoid repetition. We define macro by using macro tag.
Using macro:
Setting up Twig
Before going deep into the main features of Twig let us see how you can install Twig via Composer. If your machine does not have a Composer you can always download it from getcomposer.org. Installing the composer is just a matter of some simple clicks when you go to the website. After installing Composer run this command to install Twig:
Note: Minimum version of PHP required for Twig 2.x is PHP 7.2.5.
For Php 5.x users use the following code:
composer require 'twig/twig:~1.0'
Twig works well with both the frontend and backend of any project. So we can look at Twig from two points of view, one as Twig for template designers and the other for Twig as developers. Twig follows the snake case trend for file naming. Snake case means that every space is substituted by `_`. For example, if the file name is blog posts then it should be named as `blog_posts.html.twig`.
Why Use a PHP Templating Engine?
There are many benefits of using the Twig templating engine over basic PHP templates. Twig helps programmers to code in concise, readable, and cleaner codes. We will be seeing how Twig helps us one by one.
- Concise: Twig has a very concise syntax. Code logic and readability are not clear enough in the previous PHP templates. You can just check out a WordPress template if you do not believe it. The example code in Twig is given:
- Separation: When the project size is bigger, the separation between the UI part and the logic behind it needs to be separated. So the design architecture should separate Model, view, and controllers. However, code by designer and programmer should be merged and here the template comes into the picture. So using a template engine, like Twig, can be a plus point in this case also.
- Easy to Learn: Some developers say that PHP is itself a template engine, so why we should not learn Twig. Well, when it comes to code developers want something that is easy to learn and scalable also. Even designers also find it easy to learn and make templates in comparison to very complex languages like PHP.
- Caching: Most of the template engine provides a caching feature. It helps to work at a better speed. Twig has better performance with compiled files, which is an extra layer over caching. We have an `Environment` object which is used for loading templates. But if the `cache` option is passed with the corresponding address then Twig will cache the compile and avoid template parsing in subsequent requests. But it is just a cached version for templates. It means the template will be evaluated in all upcoming requests, but Twig has already compiled, parsed, and saved the file for you.
Code for caching the templates is:
- Filter, classifiers, and other features: The filter gives us the option to filter the information that is to be sent to the template and what should be seen to the user, in which format. Some of the filters of Twig are,
`
abs
`
,`
batch
`
,`
capitalize
`
,`
column
`
,`
convert_encoding
`
,`
country_name
`
,`
currency_name
`
,`
data_uri`
,`
date
`
,`
modify
`
, etc. There are many other filters, you can find the full list here.
- Less chance of broken code: As on one project many developers can work simultaneously, there is a high chance that one developer can disrupt the code of another developer. For solving this problem we have a version control system.
Symfony Slack Notifier
- Debug: Last but not least, debug is the most important part of any application. If we have to access all the information of a template variable, for that purpose Twig has a
`dump()`
function. We have to add`Twig_Extension_Debug`
for the`dump()`
function, at the time of creating a Twig environment.
`
dump()
`
function can be used to dump all template variable information.Reusing Templates in Twig: Three Methods
`
{% extends %}`
to allow markup sharing between templates by extending this template in another template.Now we are going to discuss three methods of reusing Twig templates.
Including Templates
In a programming language if you have some part of code that is to be used again and again then you can make a function and just call anywhere you want. The same thing happens in Twig. Suppose you have a code snippet that is performing a certain function. If you want functionality again you don’t need to rewrite that code. You can use the `include()` function to use the feature of Twig.
For example suppose the following code i used to show the profile information of user:
`
blog/_user_profile.html.twig
` ( “_”
is optional but it is recommended to have a clear distinction between the full template and template fragments.Then replace the original template with the following template to include a templating fragment.
`include()`
function is the path of the template to be included. Using the with_context
parameter you can specify the scope of variables that can be accessed by the included template.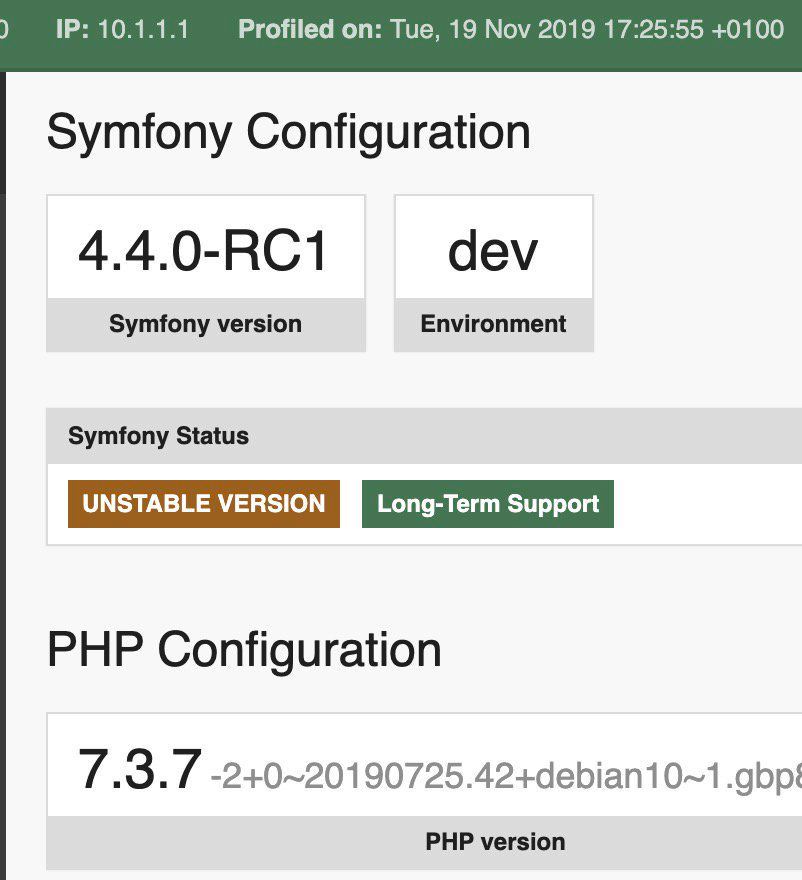
blog_post.author
variable but the included template expects it to be named as a user. In this case, you can pass an optional parameter to rename variables in the included template. Example code for this operation is below:`
with`
keyword.`only`
keyword. `ignore missing`
keywords. The `ignore missing`
is always used after the template name. Other keywords like `
only, with`
can also be used with it.Embedding Controllers
include()
function then you have to do the same database queries on every page. This method is not efficient and can have a long runtime if there is a large database.
render()
and controller()
Twig functions.First, you have to write a controller for the desired database query, here fetching the number of articles.
`
blog/_recent_articles.html.twig`
(again `_`
. This is optional but is recommended to keep template fragment and full template names different. controller()
function is given below. controller()
Twig function, controllers are accessed by special exclusive URLs to serve those template fragments. You can configure that fragment URL option as given below:Template Inheritance
As the main topic was reusing Symfony templates, the inheritance of templates must be there. In a big website or say a project there are many which are common from page to page. Header, footer, sidebar, social media bar, search bar, etc, many things are almost used on every page. It will be very inefficient and time taking if we have to write code on every page separately. Here the concept of inheritance and embedding controllers can do miracles. But if the pages share a common structure it is advised to use inheritance. Inpa bmw software download, free.
Twig follows the same inheritance process as PHP does. You have to create a parent template that can be overridden by other child templates for using different parts of the parent template.
There are three level template inheritance for simple to complex applications:
templates/base.html.twig
is used for basic elements like<head>
,<header>
,<footer>
, etc.- Inherited from
templates/base.html.twig
, templates/layout.html.twig is used for defining the structure of the content in most pages. Custom layouts can be defined usingtemplates/blog/layout.html.twig
this line - The third one which is inherited from the main page
layout.html.twig
istemplates/*.html.twig
base.html.twig
template is like this:Page sections that can not be overridden by the child templates are defined as Twig block tags.
Nuvvu naaku nachav serial. The code of blog/layout.html.twig can look like this:
blog/index.html.twig
, which will display a blog index.blog/index.html.twig
using inheritance. Each template uses its unique contents whereas the duplicate HTML structure and contents are left in the parent template.Code More Efficiently
Templating engines are always helpful when it comes to loading data directly in a website's variable at runtime. There are many templating engines and all of them have their gray areas and white areas.
Twig template provides us many features like reusing Twig templates, output escaping, debugging templates, etc. In this article, we have already seen how inheritance helps in overriding different blocks of template fragments. We have to write code once and can be used again and again. This property makes Twig unique and a great templating engine for web developers.
SymfonyCloud can trigger notifications when various events happen on a project,in any environment.
Available notifications¶
Note
At this time, only low disk space warning notification are available, butothers may be added in the future.
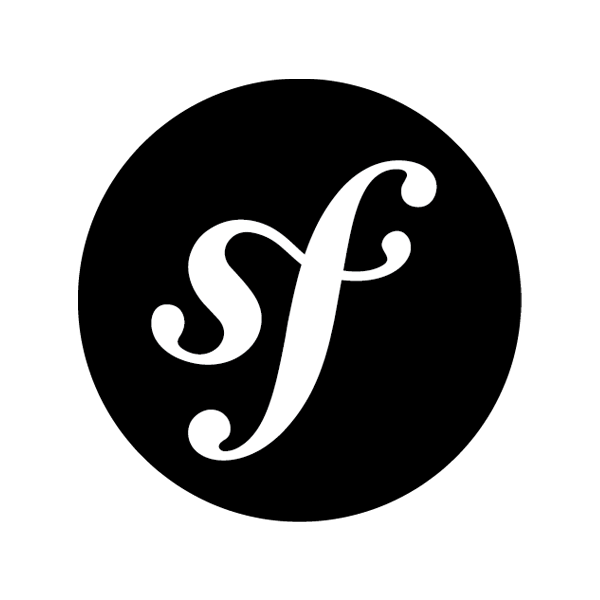
Low-disk warning¶
If any integration is configured, SymfonyCloud will monitor disk space usage onall applications and services in your cluster.
- If and when available disk space drops below 20%, a warning notification isgenerated.
- If and when available disk space drops below 10%, a critical notification isgenerated.
- If and when available disk space goes back above 20% after previously havingbeen lower, an all-clear notification is generated.
Tip
Notifications are generated every 5 minutes, so there may be a brief delaybetween when the threshold is crossed and when the notification is actuallytriggered.
Configuring notifications¶
Health notifications can be set up via the CLI through a number of differentchannels each one detailed bellow.
Email notifications¶
A notification can trigger an email to be sent, from an address of your choosingto one or more addresses of your choosing.
To do so, register a health.email
integration as follows:
Symfony Slack Monolog
The from-address
is whatever address you want the email to appear to befrom. You must specify one or more recipients
, each as its own flag. It iscompletely fine to use the same address for both from-address
andrecipients
.
Tip
You can use #admins
as a value for recipients
, this will notify everyproject administrator, removing the requirement for you to update the listwhen users changes. By default, every new project come with ahealth.email
integration setup with this value.
Caution
When using a custom from-address
, depending on the configuration of therecipient mail server (including SPF and DKIM DNS entries), the email can bemarked as spam or lost.
Slack notifications¶
A notification can trigger a message to be sent as a Slack bot. First, create anew custom bot user for your Slack groupand configure the channels you wish it to live in. Note the API Token providedby Slack.
Then register the Slack bot with SymfonyCloud using a health.slack
integration:
Symfony Slacks
That will trigger the corresponding bot to post a notification to the#channelname
channel in your Slack group.
PagerDuty notifications¶
Symfony Slack Bundle
Zinstall easy transfer free. A notification can trigger a message to be sent via PagerDuty. First, create anew PagerDuty integrationthat uses the Events API v2. Copy the “Integration Key” as known as the“routing key” for the integration.
Now register a health.pagerduty
integration:
Symfony Slacker
Any notification will now trigger an alert in PagerDuty.
